Found a Problem
The trap set up in the last post worked. The blue light was not flashing. I carefully loaded the code and “attached to running target.” The IDE showed where the code was frozen:

The code is stuck in the CaptureISR. This interrupt service routine is used to time received IR signals. Hmm. I left the IR Receiver off of the board when I put it into the case.
So, I started single stepping through the code. It was indeed stuck in the while() loop, and never coming out. That is supposed to be impossible.
There is a 4 entry FIFO (First In, First Out), so it should be drained with a maximum of 4 times through the loop, unless there is a problem. I looked at the return from the ReadStatusRegister part of the code, and it was 0xD0, or binary 11010000. Looking at the bits from the following information in the data sheet for the counter component:
uint8 Counter_ReadStatusRegister(void) Description: | This function returns the current state of the status register. |
Parameters: | None |
Return Value: | uint8: Current status register value. The status register bits are: [7]: Unused (0) [6]: FIFO not empty [5]: FIFO full [4]: Capture status [3]: Underflow status [2]: Overflow status [1]: A0 Zero status [0]: Compare output |
Reading the information on the function, the MSB should be 0 (bad information on data sheet?) ,the Fifo is Not Empty, is Not Full, A Capture occurred. For now, I will assume there is a problem other than a bad component.
So I go back to the schematic. Focusing in on the capture section, I see a possible problem. I left off the IR receiver, so there is no pull up resistor onto the Glitch Filter input, if the Pin 1[7] has a floating input.
In addition, the clock going into the GlitchFilter allows a pulse as fast as one per clock cycle, so in theory a situation could arise where the Capture Register can never be emptied by code running at 24 mhz CPU bus speed:
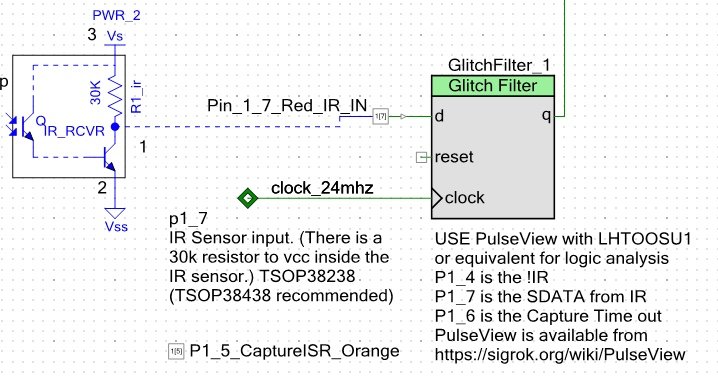
So, I take a look at the input pin’s configuration (Pin_1_7_Red_IR_IN):
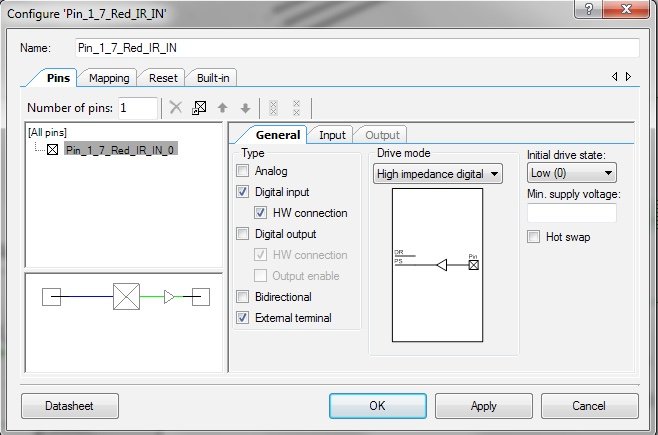
OOPS! It is high input digital (floating input). Not pulled up or pulled down. It is in the exact proper condition to generate the most amount of digital input due to noise from any source, including nearby pins. This is not a apparent problem when the IR receiver is attached, due to the 30k pull-up resistor, but, on the other hand, I did have to filter the input using a Glitch Filter, which indicates to me that pull-up may be a little too weak.
So, I configure the pin to be a “Resistive pull up” configuration (which puts an approximately 3.7k resistor on the input to VDD). I then put a note to myself in the schematic to use the pull up configuration if the IR receiver is not attached, but set for high impedance digital if attached.
This configuration is done through the “Drive mode” drop down selection box above the Pin Schematic Diagram:

Next, I went into the code and checked to see if I could “fix” a stuck FIFO so the Temperature project would continue to function properly. The first item is to prevent being stuck in a FIFO loop. So, I read the FIFO a maximum of 4 time. This allows the rest of the system to run, even though you might spend so much time in the ISR that it will appear to be extremely sluggish.
The updated code is here:
CY_ISR(CaptureISR){
P1_5_CaptureISR_Orange_Write(!P1_5_CaptureISR_Orange_Read());// toggle the isr flag
int16_t regVal=CNTR_IR_Capture_ReadStatusRegister();
if (regVal & CNTR_IR_Capture_STATUS_OVERFLOW){
capCounter=0;
return;
}
if (regVal & CNTR_IR_Capture_STATUS_CAPTURE && regVal & CNTR_IR_Capture_STATUS_FIFONEMP)
CaptureTimes[capCounter++]= CNTR_IR_Capture_ReadCapture()>>1;// divide by 2
// add failsafe to prevent being caught in while loop
// after 4 reads, leave the ISR. This handles spurious captures, to some extent
int16_t counter=4;
while (counter-- &&
CNTR_IR_Capture_ReadStatusRegister() & CNTR_IR_Capture_STATUS_FIFONEMP)
CNTR_IR_Capture_ReadCapture();
}
Reset The Trap
After recompiling and reloading, I set up the same circumstances I used for capturing this problem. The code is now loaded, and the program is running. If I have not seen any issues after a week, I will move forward with other things. I would like for it to monitor outside temperatures also. Freezing temperatures will be here soon. Hmm. Later.
Next Time, Hopefully
I will try to set up a GitHub for the FreeRTOS basic system. If that is not successful, I will upload the zipped file to this site and then start the walk through of how it works from a high level point of view.
Enjoy!